I was recently asked whether Metawidget can output JIDE components instead of standard Swing ones. The answer is yes! Metawidget makes it easy to support third-party component libraries. Here's how:
Step 1: Derive your own JideMetawidget
Subclass SwingMetawidget and override its buildActiveWidget method to select JIDE components. For example:
import static org.metawidget.inspector.InspectionResultConstants.*;
import java.util.*;
import javax.swing.*;
import org.metawidget.util.ClassUtils;
import com.jidesoft.spinner.DateSpinner;
public class JideMetawidget extends SwingMetawidget {
public JComponent buildActiveWidget(Map<String, String> attributes)
throws Exception {
String type = attributes.get( TYPE );
if ( type != null ) {
Class<?> clazz = ClassUtils.niceForName( type );
// Use JIDE DateSpinner
if (Date.class.isAssignableFrom( clazz )) {
DateSpinner dateSpinner = new DateSpinner();
dateSpinner.setFormat( "dd/MM/yyyy" );
return dateSpinner;
}
}
// Not for JIDE
return super.buildActiveWidget( attributes );
}
}
That's it! You'll get all the other Metawidget benefits (like layouts, Beans Binding, etc.) for free.
Step 2: Use JideMetawidget in your Application
To try it, download the Metawidget source and update the Swing Address Book example's ContactDialog to say...
...(on about line 135). Run the example, double click on Homer Simpson and you'll see the JIDE DateSpinner component used for the 'Date of Birth' field (previously it was just a JTextField, because vanilla Swing doesn't have a Date picker):
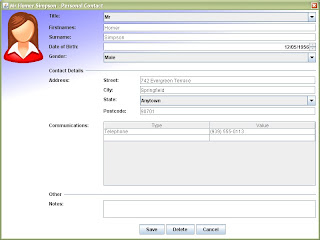
Note: JIDE's DateSpinner doesn't support null values, but
in the Address Book example Date is a nullable field. This causes errors if you click on anything other than Homer Simpson. Note that SwingX's JXDatePicker doesn't have this problem.